Hi! I’m Tomás on my last post about the LibreMesh Application (now just LimeApp). It was really fun to work with Altermundi on this project and I like the results that we achieve. I hope that everyone enjoys this post just like I enjoyed working on the application! So, let’s get started.
Finished the first part of the GSoC we started to build the next version of the app (1.0).
Resume
As a resume, the objectives for this part are:
- Do a correct use of threads trying to avoid interruptions to the user.
- To implement a new Graphical User Interface with the capability of returning messages to the user about the situation of the network (for example, could the app connect to thisnode.info? No? Why?).
- Add the app to the LibreMesh Operating System.
Fixing the HTTPGet
First things first the previous app had a bug discovered at the first meeting of the second part. If someone has a server on the address thisnode.info the connection could be reached and, in this case, the app shows that the connection to LibreMesh was correct in all the possibles cases.
So, the solution is to do an HTTPGet to thisnode.info. There’s an interesting answer in StackOverflow about this topic:
https://stackoverflow.com/questions/2793150/how-to-use-java-net-urlconnection-to-fire-and-handle-http-requests
So, we will only focus on getting an HTTPGet, so with the tools that we got from the article, I built this code:
StrictMode.ThreadPolicy policy = new StrictMode.ThreadPolicy.Builder().permitAll().build();
StrictMode.setThreadPolicy(policy);
try {
connection.getInputStream();
return true;
} catch (IOException e) {
e.printStackTrace();
return false;
}
Using threads
This code looked fine but there’s one thing that wasn’t correct according to the Android specifications. There’s a permitAll in the policy. This means that connections can happen in the principal thread of the application. To publish in the play store, we needed to change this and create a thread to run the HTTPGet, so the code now looks like this:
boolean[] success = {false};
Thread connectionThread = new Thread(new Runnable() {
public void run() {
try {
connection.getInputStream();
success[0] = true;
} catch (IOException e) {
e.printStackTrace();
return;
}
}
});
connectionThread.start();
connectionThread.join();
return success[0];
This isn’t the best example of a Thread cause doesn’t work concurrently, but it helps the app to respect the Android specification.
Thanks to our testers, we discovered that there was a problem with the latest Androids version that required too many permits to access the SSID or even the ID of the WiFi, so we decided to disable the verification that used these attributes in the latest version of Android
public static boolean verifyWifiConnection(WifiManager wm) {
if(Build.VERSION.SDK_INT <= Build.VERSION_CODES.P)
return wm.isWifiEnabled() && wm.getConnectionInfo().getNetworkId() != -1;
return wm.isWifiEnabled();
}
Also, we needed to add the attribute android:usesCleartextTraffic=”true” in the Android Manifest because the latest Android version doesn’t allow HTTP sites on WebView.
Finally, we added an error screen and changed the use of the app from three buttons to an “automatically display” of the Lime-App, or an error message.
Graphical interface
We wanted to maintain the app light, so the graphical interface needed to be only two screens:
- One with the WebView that access to the LibreMesh configuration.
- Other one with an error and some tips to fix it.
So, with these use cases, we created an error screens that looks like this:
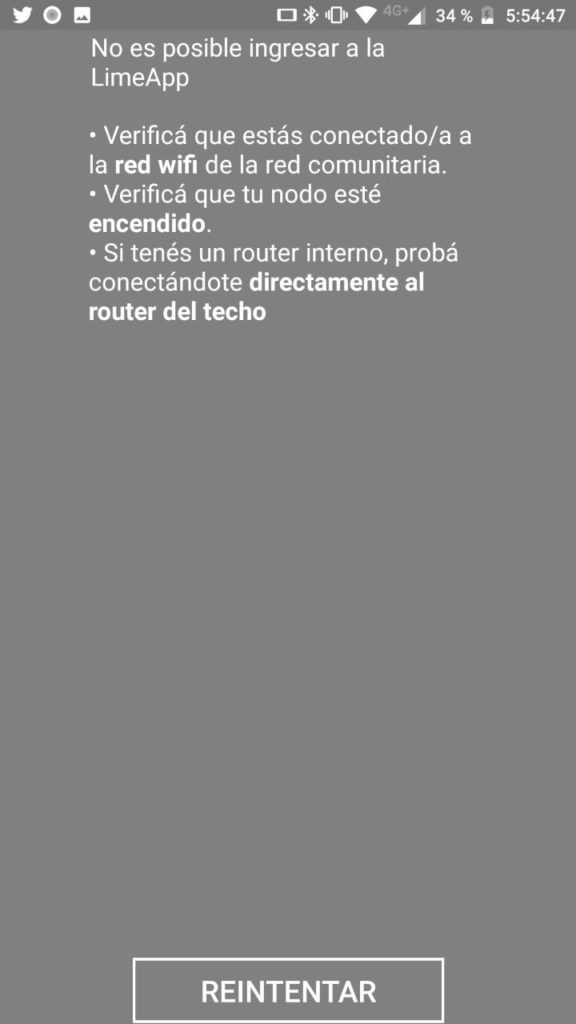
And here’s a video of how’s the app working:
Unit testing
With all the problems solved and the testers using the prerelease version, we added some unit testing using Mockito. This is an example of a Unit Test of a correct connection to the app:
@Test
public void correctConnection() {
when(wm.isWifiEnabled()).thenReturn(Boolean.TRUE);
when(wm.getConnectionInfo()).thenReturn(wi);
when(wm.getConnectionInfo().getNetworkId()).thenReturn(1);
try {
when(urlc.getInputStream()).thenReturn(null);
} catch (IOException e) {
fail();
}
MainActivity ma = new MainActivity(wm, urlc);
assertEquals(true, ma.accessToLibreMesh());
}
This test uses mocked objects to simulate a response and then it executes the function. We plan to add in the close future Integration tests.
Reducing the app size
One of the objectives that we set for the second part of the GSoC was to reduce the APK size to add the app to the LibreMesh OS. The original size was 2.91 MB and knowing that a LibreRouter has 8 MB of total space, uploading the app means to use 36% of the node space.
The first attempt to reduce the APK size was to start using the “Generate APK” function of Android Studio instead of using the debug APK. This is also needed to sign the app to publish in the Play Store. This reduced the size from 2.91 MB to 2.42 MB.
This reduction was great but isn’t enough, so we started using the tips of the ApkGolf project (https://github.com/fractalwrench/ApkGolf/b) to reduce the space. In an ideal case, we could use all of them, but we wanted to maintain things like a Constricted Layout for the Error message.
We started adding some lines to the build.gradle that add scripts that help to reduce the code and resources size.
buildTypes {
release {
minifyEnabled true
shrinkResources true
proguardFiles getDefaultProguardFile('proguard-android-optimize.txt'), 'proguard-rules.pro'
}
}
(The proguardFiles were already in the code, but also helps in the size reduction).
Another interesting setting is the resConfig. The library imports usually bring support for multiple languages. We’re currently using Spanish, so setting this to only one language reduce the size a little
resConfigs "es"
Another thing that reduces space is to convert the images to WebP (and accept a good percentage of reduction of quality). We also deleted some files and hard-coded things like the colors (to remove de .xml).
The file that occupies the most space is the one that’s related to classes (ours and imports), so the best way to reduce space use was to remove them. In the build.gradle there were a lot of imports that we added in some moment, but we removed the use of them, so remove also from the build.gradle was a good idea.
They were particularly two interesting dependencies:
implementation 'androidx.appcompat:appcompat:1.2.0'
implementation 'com.google.android.material:material:1.2.1'
The first one was added by Android Studio and we didn’t use it, but the second one was an addition to the design of the application. We removed the calls to the import and the dependency. That reduced approximately 400 KB of space.
At the end of the process, the APK size is 730,337 KB, this means a reduction of approximately 85% of space use.
Finally, we decided to publish a close version to the Play Store because all the main objectives were completed. Currently, we’re waiting for Google to finish the revision on the App.
Jekyll page
We’ve also added a Jekyll page for the project (available on Github) that shows some information about the application and the problem that solves.
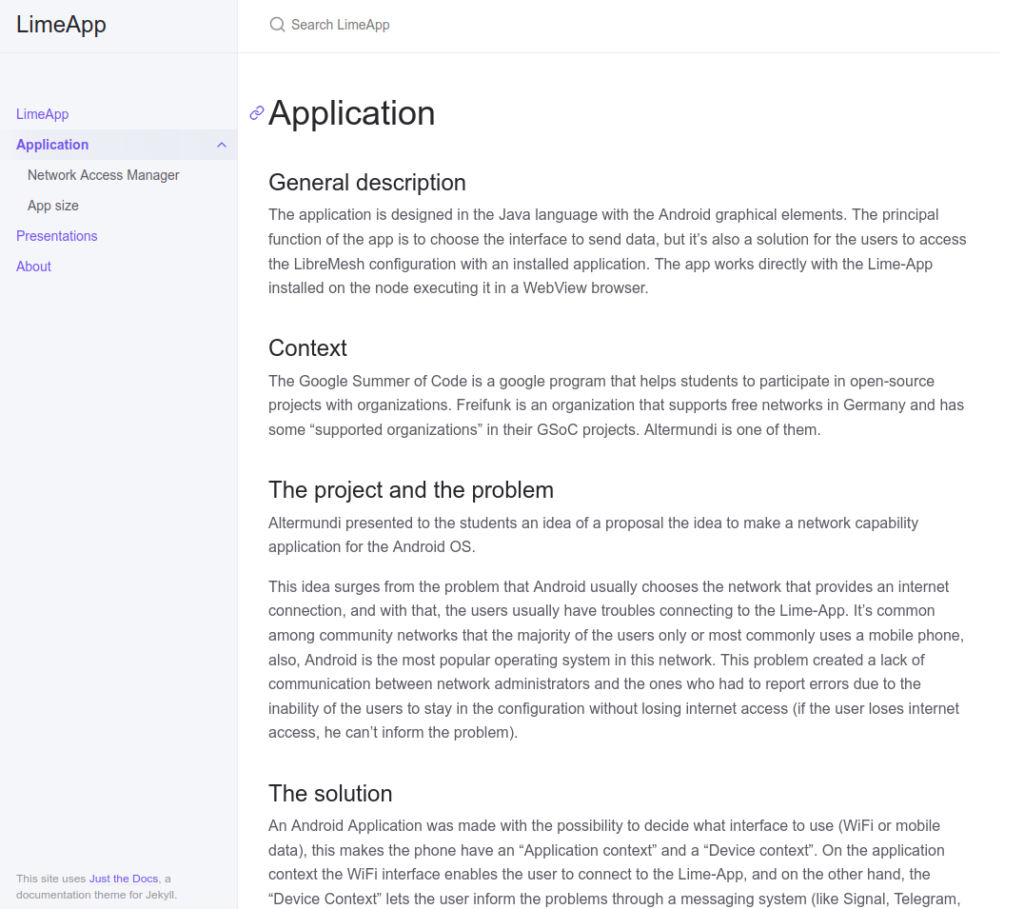
I’m really happy with the work that we did during this GSoC and I’m glad that this application is going to help Community Networks around the world. Thanks to all for letting me be part of this great community and especially to Nicolas Pace and Germán Ferrero for all the support that they gave me during this two months. Greetings and I hope that I can help with other open-source projects in the future!