Hi, Jonas here again! The first period is over and thus it’s time for an update about my GSoC’22 project ‘TX-power control in WiFi networks’. In this blogpost I will cover what I have done and achieved so far, some initial testing and evaluations, and what are the next steps for the remaining project time.
(1) Linux kernel structures for TPC
Major objective of the past weeks was to create a foundation for TPC in the Linux kernel. As I already explained in my first blog post, the Linux kernel, i.e. the mac80211
layer, has only rudimentary support for TPC per virtual interface, but not per packet or per MRR stage.
To create this foundation, it is necessary to both modify existing kernel structures and develop new structures for TX-power annotation and related information. Although the project just aims at providing TPC per packet, I decided to be more-or-less future-proof and extend this to TPC per MRR stage. There are several wifi chipsets supporting this already, and there will be more in the future.
Preliminary considerations
A major challenge when trying to implement new extensions in the network stack is the existing sk_buff
structure. This structure represents a socket buffer (SKB), meaning anything related to network packets, their control and status information, the data etc. For historical and performance reasons this structure has a fixed size, has fixed-size members and is aligned properly for cache lines. But this makes it pretty hard to introduce any extensions, e.g. new members per packet as this would violate the size-constraints and lead to either a huge performance loss or a not-working network stack. This applies to both the control path and the status path. A solution for this and how this is handled is provided in a).
Another aspect that needs to be considered are the different TPC capabilities of wifi chipsets. They often differ in:
- TPC supported? / per packet / per MRR stage
- TX-power levels and power step size
Instead of using a ‘smallest common denominator’ solution for all wifi chipsets, which would not make use of the extended capabilities of some chipsets but just a common subset for all, another approach was chosen. To achieve the best coverage of all different capabilities, the structures and annotations for TPC are designed as abstract as possible.
(a) TX-power annotation
To annotate TX-power per packet and per MRR stage, the kernel structure ieee80211_sta_rates
is used. This structure was introduced several years ago to overcome the limitations of sk_buff
and the fixed-size control buffer for each network packet. In contrast to the control buffer, this structure is not attached to each sk_buff
but rather attached to an STA and can be filled by RC algorithm and read in the TX path of a driver asynchronously. Prior to this method, the driver had to call the RC algorithm each time to retrieve the rateset for a packet.
struct ieee80211_sta_rates {
struct rcu_head rcu_head;
struct {
s8 idx;
u8 count;
u8 count_cts;
u8 count_rts;
u16 flags;
+ u8 tx_power_idx;
} rate[IEEE80211_TX_RATE_TABLE_SIZE];
};
To the internal rate array, which already allows to pass information per MRR stage, a member for the TX-power is added. The data type unsigned 8-bit
is large enough at the moment but can be easily widened if necessary.
For backwards compatibility and supporting TPC with probing, a modification of the previously mentioned control buffer per packet is also necessary. Although this does not allow an annotation per MRR stage, it is still required for compatibility reasons as some drivers still do not use the new rate table, and for probing. Probing still uses the information directly embedded into the control buffer instead of the rate table for the first rate entry / MRR stage or applies this information to the whole packet. To support this, a new member for TX-power is added to the control buffer structure:
struct ieee80211_tx_info {
...
struct {
struct ieee80211_tx_rate rates[IEEE80211_TX_MAX_RATES];
...
+ u8 tx_power_idx;
} control;
...
}
(b) Supporting different TX-power capabilities
To support different TX-power levels, ranges and step widths, the TX-power in the mac80211
layer is always specified as an index into a list of TX-power levels. This way, the mac80211
layer and RC algorithms can handle different capabilities in an abstract way, reducing code complexity and keeping possible performance effects to a minimum.
The list of supported TX-power levels is provided by the driver at time of initialization. Instead of populating a dynamically sized list with all possible TX-power levels, which would require space for each level, a driver needs to provide so-called ‘TX-power range descriptors’. A corresponding C implementation of such a descriptor for wireless TX-power was developed:
struct ieee80211_hw_tx_power_range {
u8 start_idx;
s8 start_pwr;
u16 n_levels;
s8 pwr_step;
}
With this structure, the driver can define several TX-power level ranges by specifiying an starting index, a starting power, the number of levels in this range and a step width. TX-power levels are always specified in 0.25 dBm steps to be able to define fine-grained power levels. Drivers can define several TX-power ranges with each a different step width, ascending or descending power with ascending indices, etc.
struct ieee80211_hw {
...
+ struct ieee80211_hw_tx_power_range *tx_power_ranges;
+ u8 n_power_ranges;
};
A pointer and a length indicator are added to the ieee80211_hw
structure which must be filled by a wireless driver before registering a new wifi device in the mac80211 layer.
This changes modify what was introduced by one of the commits I mentioned in my first blog post. After some implementation progress we realized, that this is much more optimal than what we initially planned to use, which was in fact a dynamically sized list containing all supported power levels.
(c) Other modifications
Some wifi chipsets do not even allow any kind of TPC, thus it should be avoided to perform calculations and keep statistics for such interfaces. To achieve this two new support flags were added. One of these also needs to be set before registering the wifi hardware to indicate TPC support. These flags are called IEEE80211_HW_SUPPORTS_TPC_PER_PACKET
and IEEE80211_HW_SUPPORTS_TPC_PER_MRR
. If both flags are not set, the driver and / or the wifi hardware does not allow or support TPC. TPC algorithms then do not need to be initialized or, in case of a joint rate and TX-power variant, the algorithm can deactivate its TX-power part.
Due to the changes that were introduced in with the commits mentioned in my first blog post, the usage of rate_info
is preferred over the usage of ieee80211_tx_rate
. But most parts of mac80211
and also most drivers still use ieee80211_tx_rate
. And there was no utility function for the conversion between ieee80211_tx_rate
and rate_info
so far. Thus, this implementation provides such an utility function for this purpose, especially as it is required in the TPC implementation in ath9k
. It is included in the mac80211
layer und thus available to all parts of the wireless stack, including other drivers.
(2) TPC support in ath9k for Atheros 802.11 a/b/g/n chipsets
To verify the implementation and to provide a first step towards TPC supported by several wireless drivers, the ath9k
wireless driver, which is responsible for Atheros 802.11 a/b/g/n AR9xxx chipsets, is extended to make use of the new mac80211
capabilities. TPC support in ath9k was already partially implemented before, but has been disabled up to now.
ath9k
has a fairly easy power range. It supports 0 dBm up to 32 dBm in 0.5 dBm steps, thus the power range is linear and the range 0 … 63 can be directly used as indices for TX-power, making it easier to set and read TX-power. This range can be easily described with a single instance of the aforementioned TX-power range descriptor. Overall seen is TPC in ath9k rather easy to implement in the control path.
A bit more challenging was the status path, especially after receiving an ACK for a packet and before the TX status is reported. For performance reasons, this is already done asynchronously. Completed SKBs are filled with information, attached to a queue and then later asynchronously dequeued and processed for TX status report. Due to the already mentioned size limitations in the SKB, TX-power could not be easily reported the same way as rates are. As a workaround, a new structure was created for this purpose but also for future extensions.
struct ath_tx_status_ext {
u8 tx_power_idx[4];
}
In the status buffer in SKB, the driver can place pointers to internal data for several purposes. Thankfully, ath9k didn’t make use of all these pointers, thus a reference to an instance of this structure can be placed in the SKB and is then available when the TX status report queue is processed. The structure can be extended in the future and has no size limitations.
(3) Setting fixed TX-power with debugfs
Appropriate structures for minstrel_ht
and a TPC algorithm have not been yet implemented as this will be part of the following weeks. To be able to already test TPC and to provide a way for setting a fixed TX-power for other purposes, minstrel_ht
was modified to already accept a fixed TX-power for all packets set via debugfs
. Following the Unix philosophy ‘everything is a file’, debugfs is a simple way for kernel modules to interact with user space applications by providing debug information or accepting parameters. Debugfs can be used like a filesystem, reading from file to get debug information or writing to a file to set e.g. a fixed rate or a fixed TX-power for wireless drivers. Many kernel modules make use of debugfs
, e.g. minstrel_ht
RC algorithm or the ath9k
driver.
The fixed TX-power set via debugfs
is written to the rate table of an STA on each update and then used by the wireless driver. minstrel_ht already uses a debugfs
-file to support fixed rate setting, thus another file is added to support the same for TX-power.
echo 63 > /sys/kernel/debug/ieee80211/phy0/rc/fixed_txpwr_idx
By writing, e.g., 63 to the file mentioned above, the TX-power will be set to the maximum possible power for ath9k
in all packets that retrieve the TX-power from minstrel_ht
. Similarly, when writing 0 to the same file, all affected packets will use the least possible TX-power. This may not be the final path of the file, there will likely be a file per STA, not per PHY, and it may be located in a slightly different subdirectory.
(4) First tests and evaluations
Some tests were already performed with an APU board with ath9k wifi chipsets acting as the access point. This is a very basic but rather realistic setup, the clients are not exactly close to the AP, there is one wall and around 3-6 meters distance between. Three clients were then used:
- iPhone 11
- Xiaomi MiR 4A
- Xiaomi Redmi AC1200
iPerf3 is used to generate traffic and measure throughput depending on the currently set TX-power. tcpdump is used on a monitor interface to measure the RSSI for all captured packets depending on the currently set TX-power. Below you can see two measurement plots of the experiments with iPhone 11 and Xiaomi MiR 4A. For the Redmi the measurement did not lead to any remarkable change in throughput.
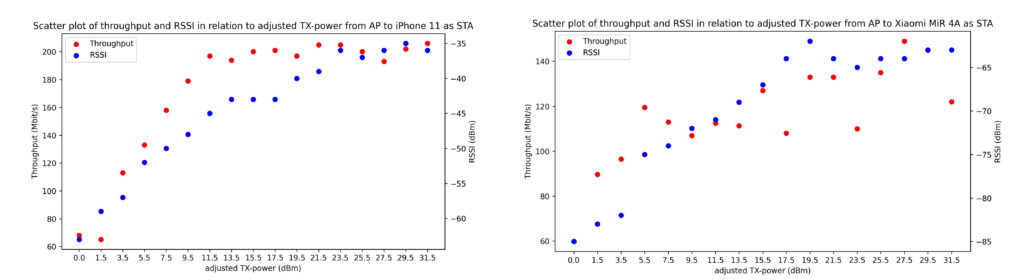
Both plots show an increase in RSSI when the TX-power is increased up to a specific point. Also the throughput usually increases, but this also depends on the actual noise, connection quality and other disturbances. Especially in case of the MiR 4A, the connection was rather bad and the throughput fluctuated more often due to instabilities, etc.
For all clients, it can be seen that after reaching a TX-power index above 45, the measured RSSI won’t increase anymore and just fluctuates due to noise. This is likely because of TX-power regulations, e.g., in Germany and many other countries the maximum TX-power for channel 36 in 5GHz band is limited to 23 dBm which would be a TX-power index of 46. Although a higher index can be set, the actual TX-power is limited by this regulatory. This will later also be included in the TX status to avoid any confusing or incorrect information.
Another observation can be made when the increasing TX-power index still leads to a higher RSSI, but the throughput stays at a level. In fact, this means that further increasing the power has no positive effect, does not lead to more throughput. It just leads to more interference in a network consisting of multiple devices and thus decreasing the overall network performance and “wasting” energy. This of course heavily depends on the capabilities of AP and STA as seen in the tests. The throughput with smaller devices, which have, e.g., less and smaller antennas, decreases much more with decreasing TX-power than with bigger device which have, e.g., more and larger antennas. For the Redmi AC1200, for example, no remarkable decrease in throughput measured could be measured while decreasing the TX-power.
(5) Conclusion and outlook
After this first period, the project achieved some remarkable progress. A mac80211 implementation with appropriate modifications and new structures was developed. By making use of this implementation in ath9k driver and performing some tests with different clients, it can be seen that it works, the TX-power can be adjusted and has an immediate effect. Also the major point of TPC could be observed: TX-power in wifi networks can be decreased for several STA without a remarkable decrease in throughput, but lower overall interference in the whole network and thus higher overall network performance.
For the second period, there are several goals:
- optimization of the mac80211 and ath9k implementations
- implementing TPC in mt76 driver for Mediatek wifi chipsets
- propose changes as patches to the Linux kernel mailing list
- testing and validation of TPC
- implementation of TPC algorithm
minstrel_ht
will be used as the starting point to implement a joint rate and TX-power control algorithm that tries to find the best rate-power combination for an STA. For this, some structures and calculations inside minstrel_ht
have to be modified. In addition, setting a fixed TX-power will be possible per STA, in the current state it is only possible to set it per interface. This requires some further adjustments to the debugfs usage inside minstrel_ht
.
As the implementation progress goes on, tests and evaluations are becoming more and more important to see whether the implementation performs as expected and which performance and/or stability gains are possible by using TPC in combination with RC. There will be extended tests with appropriate TX-power and signal measurements, also covering overall WiFi network throughput.
Thanks for reading!
One thought on “Update on TX-power control in WiFi networks – GSoC’22”