The last weeks
So it’s been a summer. Wow. It was an amazing experience taking part in this project, I feel like I have learned a lot and I hope that my work will be useful to others.
I want to take this opportunity to highlight what I set out to do and what I achieved in the end. The last weeks were probably the most satisfying bit of the entire project as modules that I had written weeks prior were slotting together seemlessly and things suddently just started to work. At the same time they were also the most frustrating with my code having two bugs that took me almost 2 weeks to finally track down.
Most of my work can be found in this repository, on the qaul_crypto branch.
I did some work on a different project, mildly related to qaul.net which might become useful soon.
Most of what I have done is backend work but there is an example application you can run. The source code compiles and has been tested under Linux (Ubuntu 14.04 and Fedora 24). By running the qaul-dbg binary (under src/client/dbg/qaul-dbg you will create two users (to emulate a communication between two nodes), add their public keys to a keystore and then exchange and verify a signed message. Feel free to play around with the code for this demo, that can be found under src/qaullib/qcry_wrapper.c.
In this final blog post I will talk a bit about what I have done, what it means for the project and how my ~2.2k lines of c-code contribution will affect the future of qaul.net.
What I have done
The basic idea behind my project was to provide a cryptography submodule to qaul.net to make it a safer and more verifiable place to communicate in. Part of that are of course ways to sign and verify messages but at the same time a core aspect of that are also user identities.
The two biggest parts of the crypto module are internally referenced as qcry_arbit and qcry_context. As I have already explained in previous blog posts, those are the Arbiter (which provides a static API to use in the rest of the library) and the Context which holds the actual “magic” of signatures, identities, keys and targets. In an earlier diagram there was a dispatcher. However due to testing the functions, it was deemed “overkill” and the functionality was integrated into the arbiter, meaning less abstraction layers and more flat code (which is always easier to understand and maintain).
With the arbiter in place it is now possible to create unique users with a unique fingerprint that can be shared on the network to identify people as well as sign and verify messages. What has also been done is provide new message types for OLSR (the routing protocoll used in qaul.net) to propagate this new information among nodes.
What I haven’t yet done
Unfortunately there were some delays due to problems with the main crypto library in use in the project as well as a few bugs that really slowed down progress in the last few weeks. Due to that the entire encryption part has been delayed. There are functions planned and partially provided to take care of these tasks however no code behind them yet to implement it.
The feature is currently in planning and will land in a later patch, probably after the initial merge of the module has gone through as there are a lot of things to be taken care of.
Current state
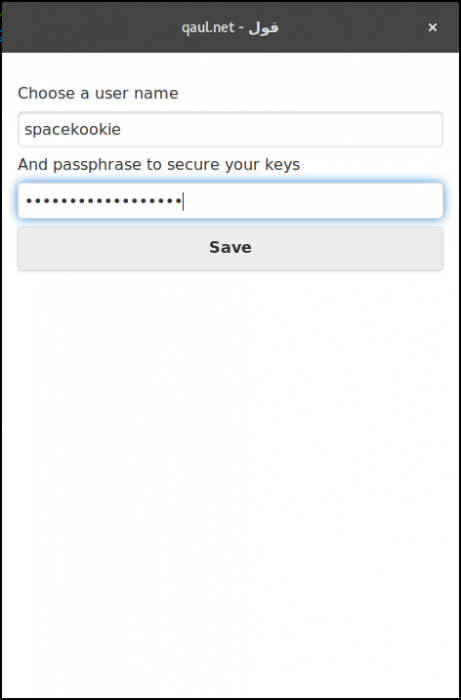
As said above, it is currently possible to create users, sign and verify messages and to flood the users fingerprint as well as public key to other users. However this doesn’t address a few problems that we have had while integrating the arbiter API into the rest of libqaul. Due to technical limitations in the communication system it’s currently not possible to sign private messages. This is due to how the communication subsystem works; which is currently being redesigned from scratch.
This means that any merge that will go through now will need to be adjusted to work with a new communication backend in a few weeks. As such, it is a bit unclear when exactly and how the crypto code will be merged. I am looking forward to working with the team on resolving these issues. And am also looking forward to a new shiny communication backend. But for now it means that merging the code I’ve written for the Summer of Code might be difficult.
New message types were added as well to provide a way to exchange these new payloads (signatures, public keys, fingerprints, etc) without breaking backwards compatibility with older nodes. What happens is that new nodes send out both a new and an old hello message. Other new nodes ignore the old hello message and can address the new node via it’s fingerprint and use verifiable channels of communication while old nodes are oblivious to any change. Until they are updated π
In conclusion
The Google Summer of Code brought qaul.net a very big step closer to being a more safe and secure place to communicate on. The crypto submodule is tested and easy to use. What might happen is that parts of the code get merged (the crypto submodule itself) without merging any of the code that hooks into the communication stack.
There are still things to be optimisised, probably some hidden bugs to be fixed and there definately is some work needed to make private messaging actually private.
This might be the end of the Summer of Code and a “final evaluation” for this part of the project but I am by no means done. I was interested in open source software before, wrote my own projects, contributed small chunks of code to other people. But this is the first time I have been deeply involved in a large community project and the first time I learned to deal with integrating with other peoples code. And it is very largely due to the Summer of Code, Freifunk and my mentor.
I had a lot of fun working on this project and I am looking forward to more contributions. Even if it was frustrating and very stressfuly at times I’m glad to have participated. The experience tought me a lot about communication in teams, software development and funnily enough signature alignments π
Acknowledgements
First of all I want to thank my mentor Mathias Jud to have introduced me to qaul.net and the Google Summer of Code. I also want to thank him for his support and guidance with problems I had while interfacing the arbiter with the rest of libqaul.
In addition I want to thank saces, another member of the qaul.net team who helped me in resolving dependency issues as well as problems I caused in the build system which prevented my code from building on Windows (woops).
Lastly want to thank Freifunk for providing me with this opportunity as well as Google for organising and sponsoring the Summer of Code projects.